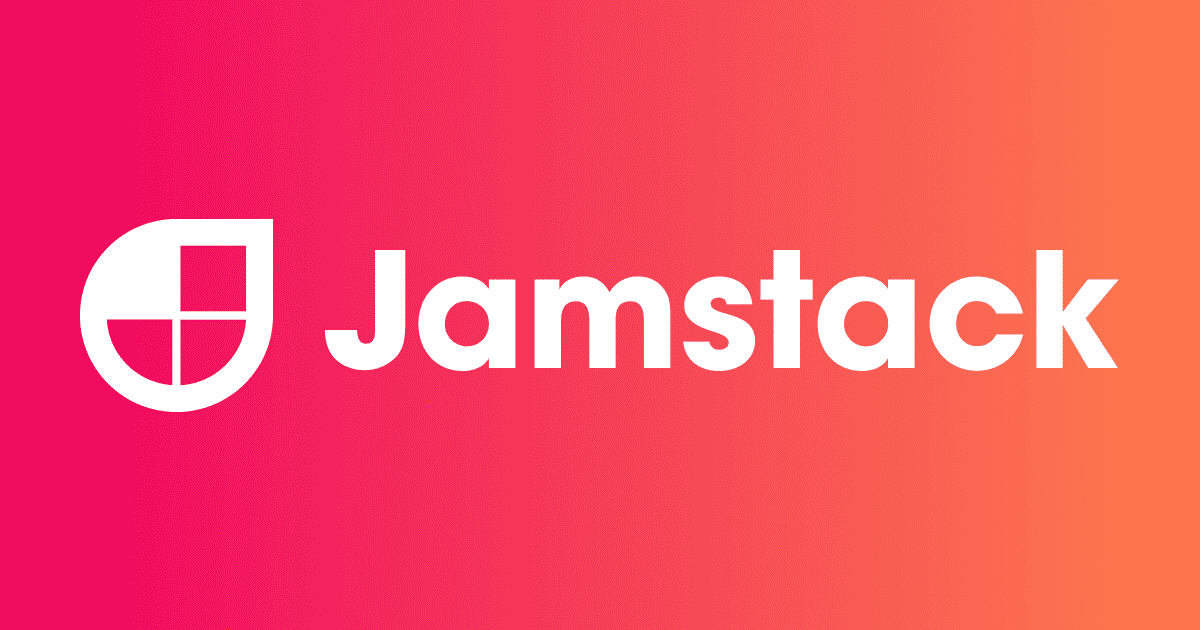
How to fix strapi error 403 and 500 on post requests
Hi guys, today we will fix some common errors you might experience when trying to make a POST request to strapi and the content-type you are posting to has related fields like category, tags, etc.
I am currently working on my personal project "Safe Haven", a JAMSTACK real estate website. I am using Next JS for the Frontend, and Strapi for the Backend. In the project, I want users to be able to add their own ads. Thereby, making POST requests to strapi.
I encountered these two errors; 403 bad request and 500 internal server errors. In this post I will show you how I fixed the errors.
Before we dive into the frontend, let's open our strapi directory and make sure we have our controller coded correctly. In my project, I am making requests to the adverts
content-type.
Goto your strapi root directory/api/advert/controller/advert.js
My content-type is advert
yours will be different. Just locate the .js file and open it. Add the below code to the controller and edit it according to your content type
"use strict";
/**
* Read the documentation (https://strapi.io/documentation/v3.x/concepts/controllers.html#core-controllers)
* to customize this controller
*/
const { parseMultipartData, sanitizeEntity } = require("strapi-utils");
module.exports = {
async create(ctx) {
let entity;
if (ctx.is("multipart")) {
const { data, files } = parseMultipartData(ctx);
data.user = ctx.state.user.id;
entity = await strapi.services.advert.create(data, { files });
} else {
ctx.request.body.user = ctx.state.user.id;
entity = await strapi.services.advert.create(ctx.request.body);
}
return sanitizeEntity(entity, { model: strapi.models.advert });
}
};
Now in the frontend of our app (I am using Next JS), create your form fields, and let's head straight to posting the data to strapi, In my project, I am using React hook form and Zod for the form.
The above diagram is the response from strapi when I make a GET request to /adverts
. We can see the "category" and "userspermissionsuser" fields are objects, they are related types to the advert content type. Hence updating these fields require passing an object that will contain all the fields within the object. For example, the category field below
If we post to only category.name we will get a 403 bad request error, and if we fail to update every field in the category object, we will get 500 internal server error.
How I fixed this
To fix this, all we have to do is send the data in the category field. In my project, I have 3 categories (Buy, Rent, and Shortlet). I am going to store their objects in separate variables.
const buyCategory = {
_id: '6202f6ba2bf36d19hfhf805b16e6',
filterOptions: null,
name: 'Buy',
published_at: '2022-02-08T23:03:30.407Z',
createdAt: '2022-02-08T23:03:22.048Z',
updatedAt: '2022-02-08T23:03:31.166Z',
__v: 0,
id: '6202f6ba2bf36d19805bhf16e6',
};
const rentCategory = {
_id: '6202f6d02dfsfbf36d19805bfd16e7',
filterOptions: null,
name: 'Rent',
published_at: '2022-02-08T23:03:52.916Z',
createdAt: '2022-02-08T23:03:44.996Z',
updatedAt: '2022-02-08T23:03:53.651Z',
__v: 0,
id: '6202f6d0fsdfs2bf36d19805b16e7',
};
const shortletCategory = {
_id: '6202f6e52bf36d19805bfsdf16e8',
filterOptions: null,
name: 'Shortlet',
published_at: '2022-02-08T23:04:13.984Z',
createdAt: '2022-02-08T23:04:05.970Z',
updatedAt: '2022-02-08T23:04:14.725Z',
__v: 0,
id: '6202f6e52bf36d19805b16edsfd8',
};
Now before we post to strapi we will do a little check to know the selected category, and add the selected category to our query
const onSubmit = handleSubmit((data) => {
const categorySelected =
data.category === 'Buy'
? buyCategory
: data.category === 'Rent'
? rentCategory
: shortletCategory;
axios
.post(
`url/adverts`,
{
title: data.title,
category: categorySelected,
price: data.price,
users_permissions_user: user,
},
{
headers: {
Authorization: `Bearer ${user.jwt}`,
},
}
)
.then(() => {})
.catch(() => {});
});
Make sure to add the user to the request else it will return an "unauthorized error".
I hope this is helpful. kindly like and share this post on your timeline 🤝
Tags: